SkyProj Projections
SkyProj supports a wide variety of map projections, primarily equal-area.
The fundamental base projection class is the skyproj.Skyproj()
sky projection.
This class is a container for a number of elements.
In particular, it contains:
A coordinate reference system (CRS) (a subclass of
skyproj.SkyCRS()
) which describes a given PROJ projection. Theskyproj.SkyCRS()
conforms to the matplotlibprojection
interface.A matplotlib axis (
skyproj.SkyAxes()
) to handle general plotting.A matplotlib axis artist (
mpl_toolkits.axisartist.Axes()
) for drawing gridlines and labels.A large number of methods for drawing maps, lines, polygons, and colorbars.
When initializing any skyproj.Skyproj()
object, there are a number of parameters that can be set.
The most common options that a user would want to specify are:
ax
: The specific axis object to replace with the skyproj axis. If not specified, it will use the current axis as determined by matplotlib.lon_0
: The central longitude of the projection. For most pseudocylindrical projections, the distortion will be smallest near the central longitude.lat_0
: The central latitude of the projection. Onlyskyproj.LaeaSkyproj()
andskyproj.GnomonicSkyproj()
supportlat_0
.extent
: Default extent of the map, specified as[lon_min, lon_max, lat_min, lat_max]
. This may be overridden if a map is plotted withzoom=True
.autorescale
: If this is set then the color bars will automatically rescale when the map is zoomed. This can be useful for maps with wide dynamic range.
Cylindrical/Plate carrée
The default sky projection is the cylindrical Plate carrée projection. This projection is convenient because it is a straight mapping from longitude/latitude to x/y. However, this projection is not very useful for plotting data due to the large distortions. The red circles are Tissot indicatrices, which visualize the local distortions of the map projection.
The cylindrical Plate carrée projection can be accessed with skyproj.Skyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.Skyproj(ax=ax)
sp.tissot_indicatrices()
plt.show()
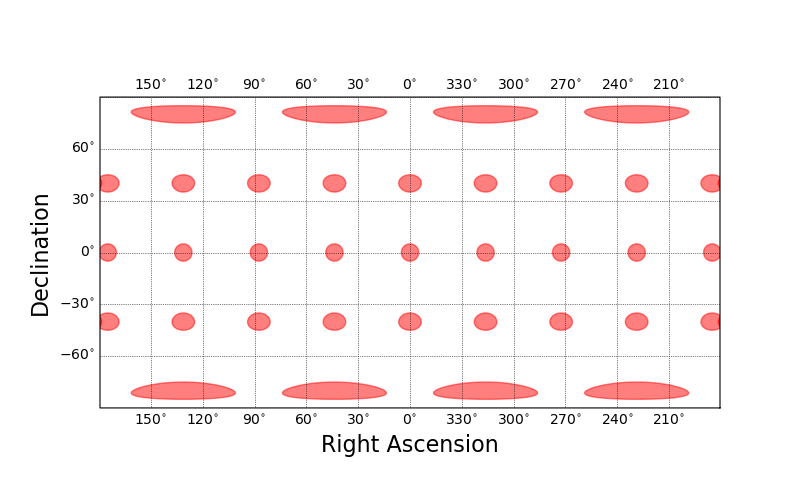
McBrydeSkyproj
The McBryde-Thomas Flat Polar Quartic projection is an equal-area pseudocylindrical projection that has been used for the Dark Energy Survey.
The McBryde projection can be accessed with skyproj.McBrydeSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.McBrydeSkyproj(ax=ax)
sp.tissot_indicatrices()
plt.show()
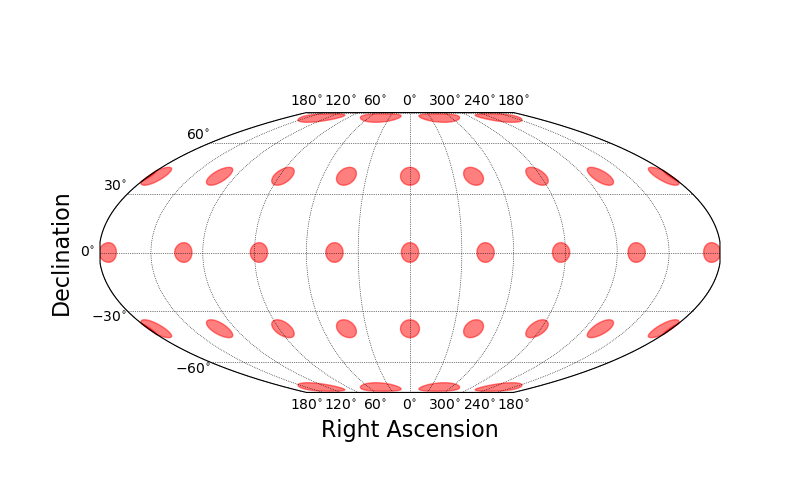
LaeaSkyproj
The Lambert Azimuthal Equal Area projection is an equal-area projection that is a good choice when focusing on the South or North pole. The distortion at the edges make it a poor choice for full sky mapping.
The Lambert Azimuthal Equal Area projection has one additional parameters beyond the defaults.
This is lat_0
, which allows you to shift the latitude of the center of the projection as well as the longitude (with lon_0
).
The Lambert Azimuthal Equal Area projection can be accessed with skyproj.LaeaSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.LaeaSkyproj(ax=ax, lat_0=-90.0)
sp.tissot_indicatrices()
plt.show()
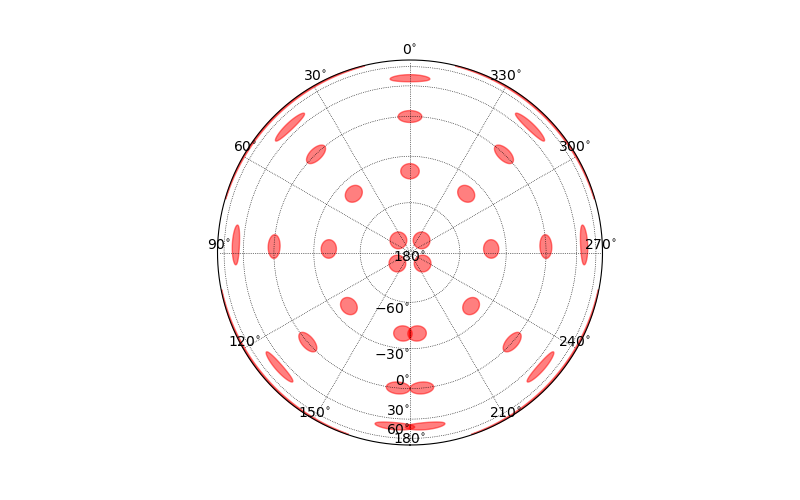
MollweideSkyproj
The Mollweide projection is an equal-area pseudocylindrical projection that is popular for astronomical all-sky maps. Due to the ambiguity of labels at the poles, the default is to put the Right Ascension labels along the equator.
The Mollweide projection can be accessed with skyproj.MollweideSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.MollweideSkyproj(ax=ax)
sp.tissot_indicatrices()
plt.show()
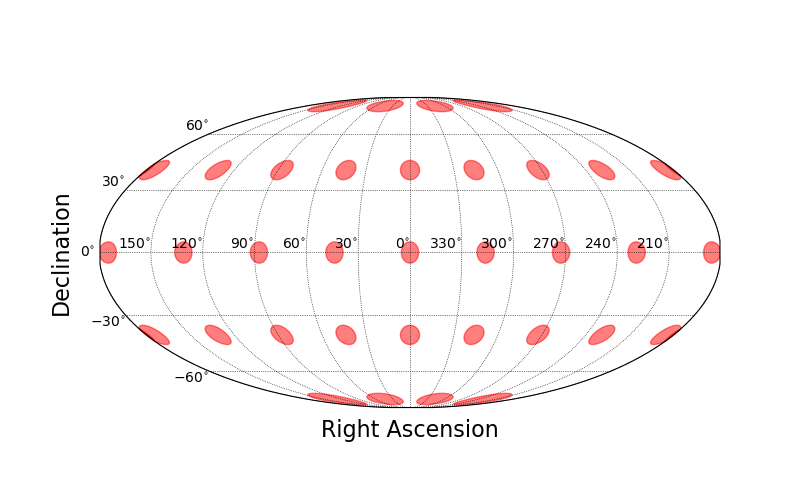
HammerSkyproj
The Hammer-Aitoff projection is an equal-area projection designed to have less distortion than the Mollweide projection. Due to the ambiguity of labels at the poles, the default is to put the Right Ascension labels along the equator.
The Hammer-Aitoff projection can be accessed with skyproj.HammerSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.HammerSkyproj(ax=ax)
sp.tissot_indicatrices()
plt.show()
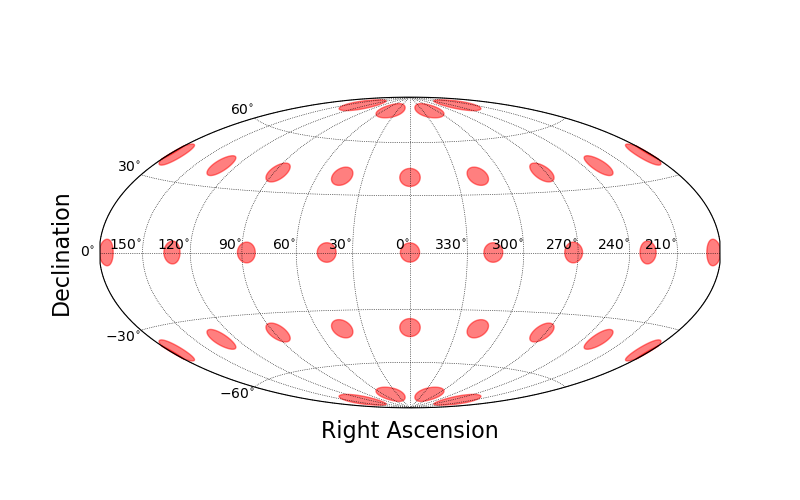
EqualEarthSkyproj
The Equal Earth projection is an equal-area pseudocylindrical projection designed for easy computability and reasonably accurate shapes.
The Equal Earth projection can be accessed with skyproj.EqualEarthSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.EqualEarthSkyproj(ax=ax)
sp.tissot_indicatrices()
plt.show()
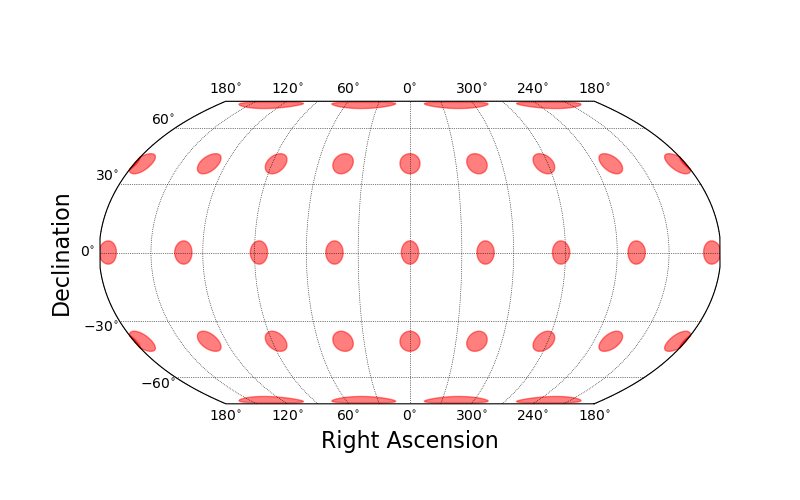
GnomonicSkyproj
The Gnomonic projection is the tangent-plane map projection that displays all great circles as lines. It is only possible to display less than half the sphere in this projection, so is not available for full-sky mapping. No distortion occurs at the arbitrary tangent point, and thus this projection is used for close-in zooms of small regions. When reprojecting small zooms, SkyProj will use the Gnomonic projection.
The Gnomonic projection has one additional parameters beyond the defaults.
This is lat_0
, which allows you to shift the latitude of the center of the projection as well as the longitude (with lon_0
).
The default projection range is a square 1 degree by 1 degree centered at the tangent point.
The Gnomonic projection can be accessed with skyproj.GnomonicSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.GnomonicSkyproj(ax=ax, lon_0=50.0, lat_0=60.0)
sp.ax.circle(50.0, 60.0, 0.25, fill=True, color='red', alpha=0.5)
plt.show()
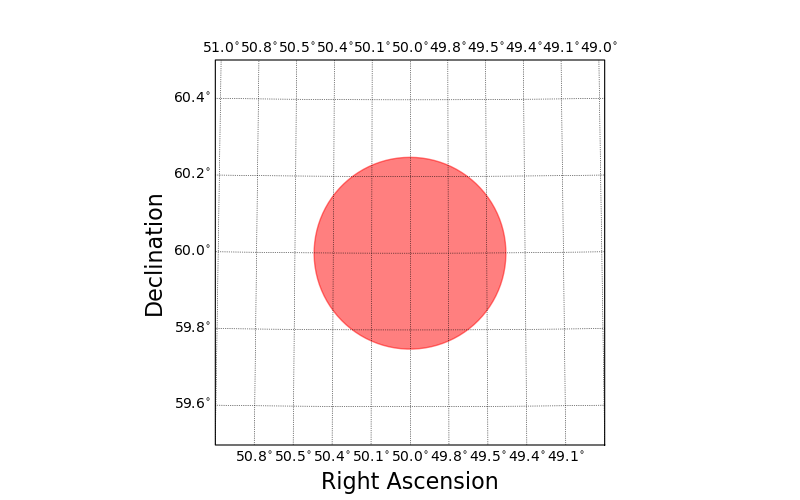
ObliqueMollweideSkyproj
The Oblique Mollweide projection is a version of the Mollweide projection such that the poles have been rotated. Support for Oblique Mollweide in SkyProj is experimental, and may not yield attractive results in all cases.
The Oblique Mollweide projection has two additional parameters beyond the defaults.
These are lon_p
and lat_p
, the longitude and latitude of the North Pole in the unrotated coordinate system.
The Oblique Mollweide projection can be accessed with the skyproj.ObliqueMollweideSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.ObliqueMollweideSkyproj(ax=ax, lat_p=45.0, lon_p=-90.0)
plt.show()
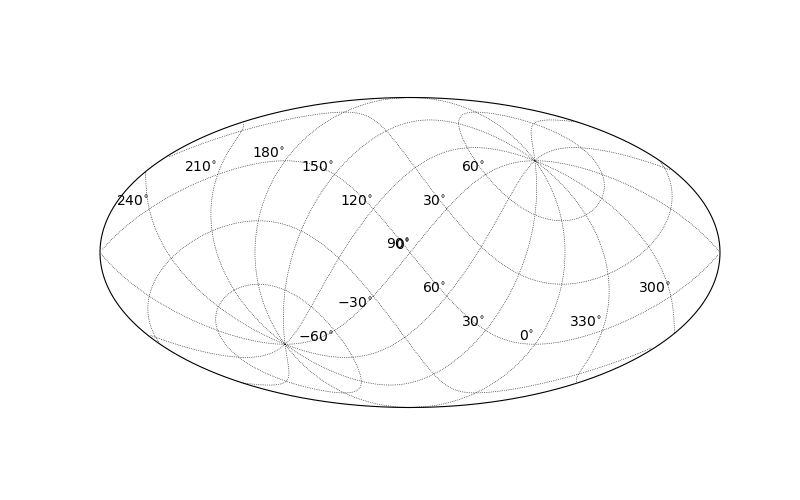
AlbersSkyproj
The Albers Equal Area projection is a conic, equal area projection that provides minimum distortion between two (configurable) parallels, at the expense of large distortion away from these parallels. The Albers Equal Area projection does not provide very attractive maps over the full sky because of the distortions, but it does work for areas as large as the Dark Energy Survey footprint.
The Albers Equal Area projection has two additional parameters beyond the defaults.
These are lat_1
and lat_2
, the latitude of the two parallels to define the projection.
These absolute values of these parallels must not be equal.
The Albers Equal Area projection can be accessed with the skyproj.AlbersSkyproj()
class.
import matplotlib.pyplot as plt
import skyproj
fig, ax = plt.subplots(figsize=(8, 5))
sp = skyproj.AlbersSkyproj(ax=ax, lat_1=15.0, lat_2=45.0)
sp.tissot_indicatrices()
plt.show()
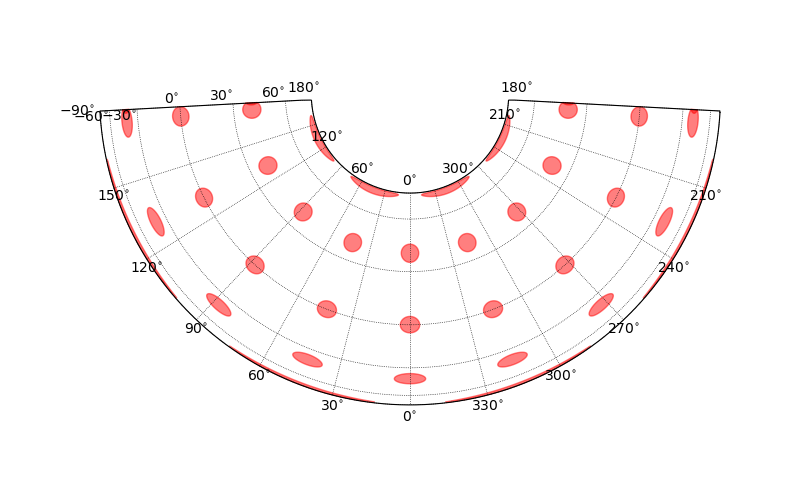